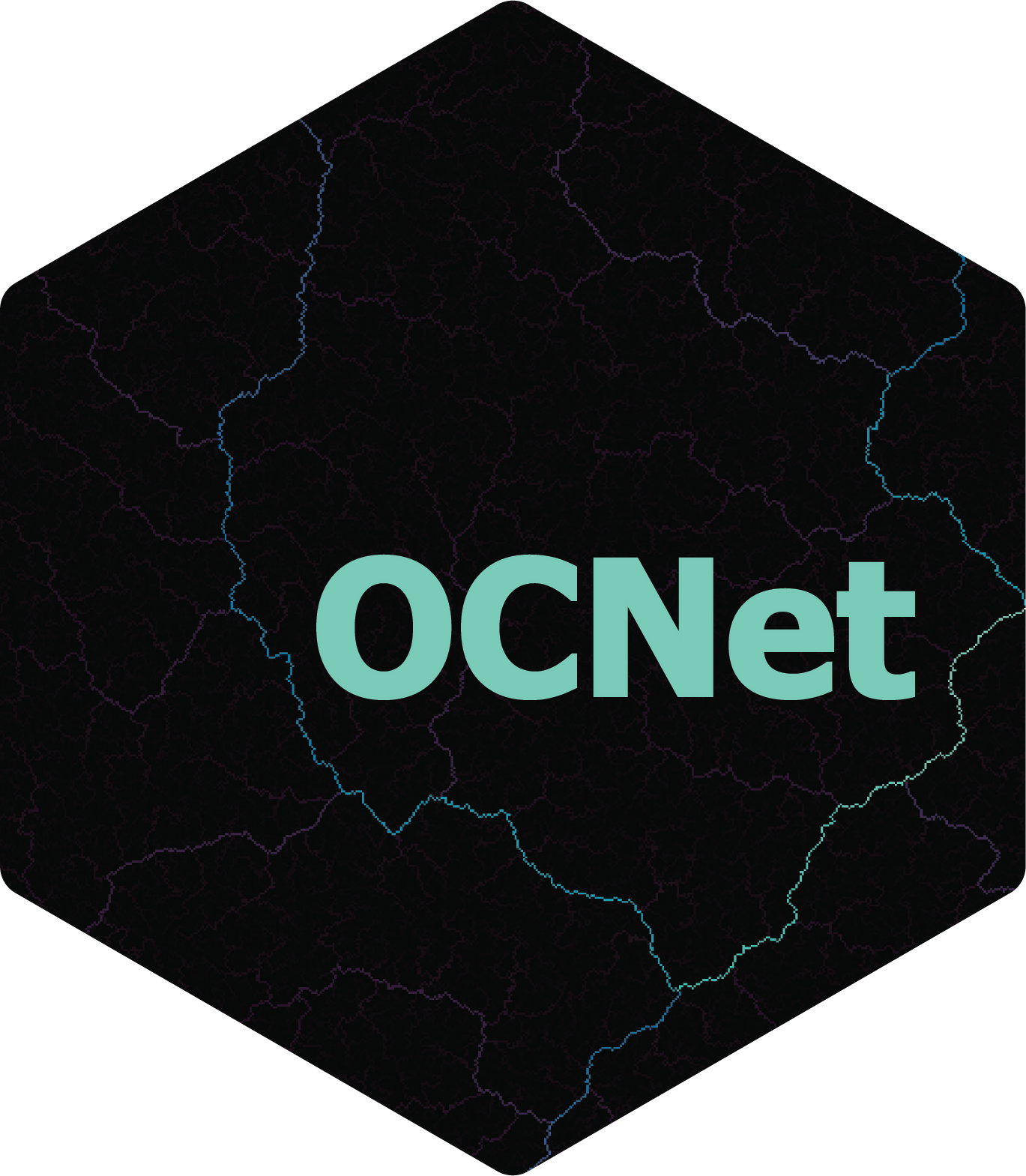
Generate 3D landscape from an Optimal Channel Network
landscape_OCN.Rd
Function that calculates the elevation field generated by the OCN and the partition of the domain into different catchments.
Arguments
- OCN
A
river
object as produced bycreate_OCN
.- slope0
slope of the outlet pixel (in elevation units/planar units).
- zMin
Elevation of the lowest pixel (in elevation units).
- optimizeDZ
If
TRUE
, when there are multiple catchments, minimize differences in elevation at the catchment borders by lifting catchments, while respectingzMin
. IfFALSE
, all outlet pixels have elevation equal tozMin
. This option is not effective for OCNs generated viacreate_general_contour_OCN
.- optimMethod
Optimization method used by function
optim
(only used ifoptimizeDZ = TRUE
).- optimControl
List of control parameters used by function
optim
(only used ifoptimizeDZ = TRUE
).- displayUpdates
State if updates are printed on the console while
landscape_OCN
runs.0
No update is given.
1
Concise updates are given.
2
More extensive updates are given (this might slow down the total function runtime).
Note that the display of updates during optimization of elevations (when
optimizeDZ = TRUE
) is controlled by parameteroptimControl$trace
.
Value
A river
object that contains all objects contained in OCN
, in addition to the objects listed below.
A new sublist CM
, containing variables at the catchment aggregation levels, is created.
FD$slope
Vector (of length
OCN$FD$nNodes
) of slope values (in elevation units/planar units) for each FD pixel, as derived by the slope/area relationship.FD$leng
Vector (of length
OCN$FD$nNodes
) of pixel lengths.OCN$FD$leng[i] = OCN$FD$cellsize
if flow direction ini
is horizontal or vertical;OCN$FD$leng[i] = OCN$FD$cellsize*sqrt(2)
if flow direction ini
is diagonal.FD$toCM
Vector (of length
OCN$FD$nNodes
) with catchment index values for each FD pixel. Example:OCN$FD$toCM[i] = j
if pixeli
drains into the outlet whose location is defined byoutletSide[j]
,outletPos[j]
.FD$XDraw
When
periodicBoundaries = TRUE
, it is a vector (of lengthOCN$FD$nNodes
) with real X coordinate of FD pixels. IfperiodicBoundaries = FALSE
, it is equal toOCN$FD$X
.FD$YDraw
When
periodicBoundaries = TRUE
, it is a vector (of lengthOCN$FD$nNodes
) with real Y coordinate of FD pixels. IfperiodicBoundaries = FALSE
, it is equal toOCN$FD$Y
.FD$Z
Vector (of length
OCN$FD$nNodes
) of elevation values for each FD pixel. Values are calculated by consecutive implementation of the slope/area relationship along upstream paths.CM$A
Vector (of length
OCN$nOutlet
) with values of drainage area (in square planar units) for each of the catchments identified by the correspondingOCN$FD$outlet
.CM$W
Adjacency matrix (
OCN$nOutlet
byOCN$nOutlet
) at the catchment level. Two catchments are connected if they share a border. Note that this is not a flow connection. Unlike the adjacency matrices at levels FD, RN, AG, this matrix is symmetric. It is aspam
object.CM$XContour
(CM$Y_contour
)List with number of objects equal to
OCN$FD$nOutlet
. Each objecti
is a list with X (Y) coordinates of the contour of catchmenti
for use in plots withexactDraw = FALSE
(see functionsdraw_contour_OCN
,draw_thematic_OCN
). If catchmenti
is constituted by regions that are only connected through a diagonal flow direction,CM$XContour[[i]]
(CM$Y_contour[[i]]
) contains as many objects as the number of regions into which catchmenti
is split.CM$XContourDraw
(CM$YContourDraw
)List with number of objects equal to
OCN$FD$nOutlet
. Each objecti
is a list with X (Y) coordinates of the contour of catchmenti
for use in plots withexactDraw = TRUE
(see functionsdraw_contour_OCN
,draw_thematic_OCN
). If catchmenti
is constituted by regions that are only connected through a diagonal flow direction,CM$XContourDraw[[i]]
(CM$YContourDraw[[i]]
) contains as many objects as the number of regions into which catchmenti
is split.OptList
List of output parameters produced by the optimization function
optim
(only present ifoptimizeDZ = TRUE
).
Finally, slope0
and zMin
are passed to the river
as they were included in the input.
Details
The function features an algorithm (which can be activated via the optional input optimizeDZ
) that, given the network
configuration and a slope0
value, finds the elevation of OCN$nOutlet - 1
outlets relative to the elevation of the first
outlet in vectors outletSide
, outletPos
such that the sum of the absolute differences elevation of neighboring pixels
belonging to different catchments is minimized. Such objective function is minimized by means of function optim
.
The absolute elevation of the outlet pixels (and, consequently, of the whole lattice) is finally attributed by imposing
OCN$FD$Z >= zMin
. Note that, due to the high dimensionality of the problem, convergence of the
optimization algorithm is not guaranteed for large OCN$nOutlet
(say, OCN$nOutlet > 10
).
Examples
# 1) draw 2D elevation map of a 20x20 OCN with default options
OCN2 <- landscape_OCN(OCN_20)
if (FALSE) {
# 2) generate a 100x50 OCN; assume that the pixel resolution is 200 m
# (the total catchment area is 20 km2)
set.seed(1)
OCN <- create_OCN(100, 50, cellsize = 200,
displayUpdates = 0) # this takes about 40 s
# use landscape_OCN to derive the 3D landscape subsumed by the OCN
# by assuming that the elevation and slope at the outlet are 200 m
# and 0.0075, respectively
OCN <- landscape_OCN(OCN, zMin = 200, slope0 = 0.0075)
# draw 2D and 3D representations of the landscape
draw_elev2D_OCN(OCN)
draw_elev3D_OCN(OCN)
draw_elev3Drgl_OCN(OCN)
}
if (FALSE) {
# 3) generate a 100x50 OCN with 4 outlets
set.seed(1)
OCN <- create_OCN(100, 50, cellsize = 200,
nOutlet = 4, displayUpdates = 0) # this takes about 40 s
# use landscape_OCN and optimize elevation of outlets
OCN <- landscape_OCN(OCN, slope0 = 0.0075,
optimizeDZ = TRUE)
# display elevation of outlets and 2D elevation map
OCN$FD$Z[OCN$FD$outlet]
draw_elev2D_OCN(OCN)
}